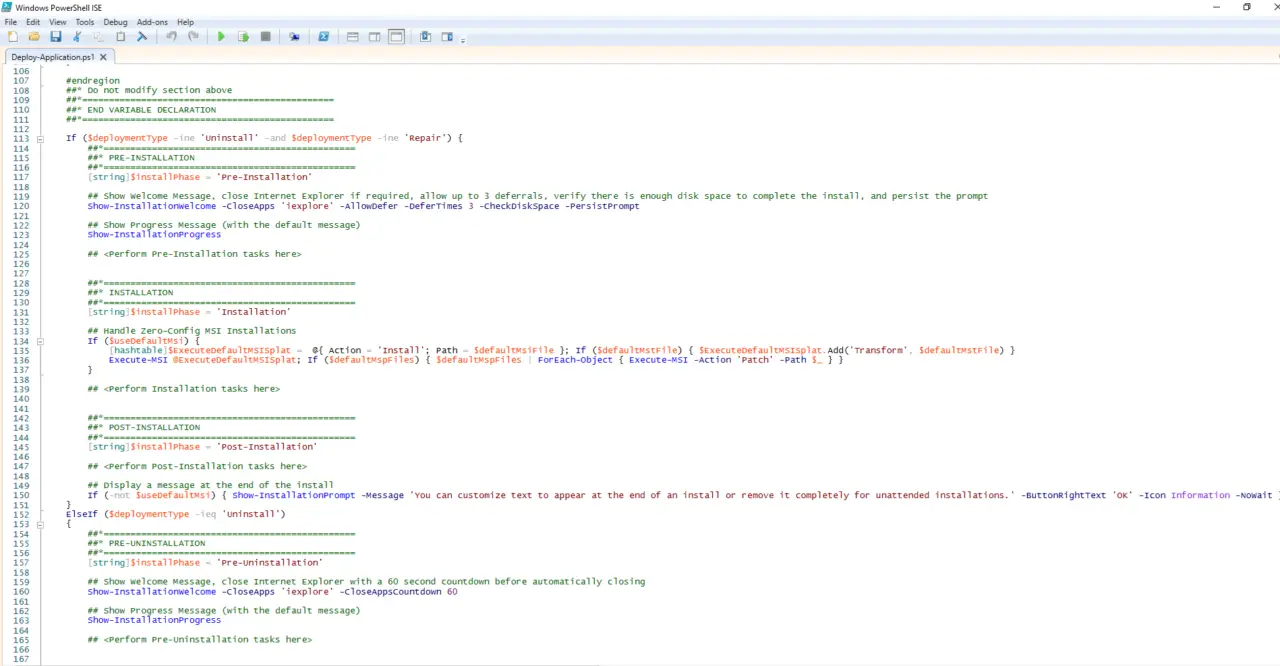
This article will serve as an informative guide and give you a clear understanding of how to install & set CMTrace as the default log viewer for all users using the Powershell App Deployment Toolkit. The PowerShell App Deployment Toolkit can be used to replace your WiseScript, VBScript and Batch wrapper scripts with one versatile, re-usable and extensible tool. This tool is an absolute must for Microsoft Endpoint Manager Configuration Manager (MEMCM) / (SCCM) Administrators or anyone who is responsible for packaging and deploying software.
How to Install & Set CMTrace as Default Log Viewer for All Users Using the PowerShell App Deployment Toolkit
- Download the Powershell App Deployment Toolkit 3.8.4:
- Download the zip file to a folder created at (C:\Downloads)
- Open Windows PowerShell by Right-Clicking on Windows PowerShell and selecting Run as Administrator
- Enter the following command to remove the Zone.Identifier:
Unblock-File -Path C:\Downloads\PSAppDeployToolkit_v3.8.4.zip
- Enter the following command to extract the contents of the zip file:
Expand-Archive -Path C:\Downloads\PSAppDeployToolkit_v3.8.4.zip -DestinationPath C:\Downloads\PADT
- Enter the following commands to copy the AppDeployToolkit & Files folder to “C:\Downloads\CMTrace“:
Copy-Item -Path "C:\Downloads\PADT\Toolkit\AppDeployToolkit" -Destination "C:\Downloads\CMTrace\AppDeployToolkit" -Recurse
Copy-Item -Path "C:\Downloads\PADT\Toolkit\Files" -Destination "C:\Downloads\CMTrace\Files"
You should now see the AppDeploymentToolkit folder with files & the empty Files folder at “C:\Downloads\CMTrace”
Next we’ll want to the locate the stand alone EXE for CMTrace.
- Option 1: Indentify possible CMTrace.exe locations in this article
- https://docs.microsoft.com/en-us/mem/configmgr/core/support/cmtrace
- Copy the CMTrace.exe to “C:\Downloads\CMTrace\Files”
- OR
- Option 2: Copy the CMTrace.exe from the Configuration Manager Toolkit
- Navigate to: https://www.microsoft.com/en-us/download/details.aspx?id=50012
- Download & Install the System Center 2012 R2 Configuration Manager Toolkit
- Navigate to: “%ProgramFiles(x86)%\ConfigMgr 2012 Toolkit R2\ClientTools”
- Copy the CMTrace.exe to “C:\Downloads\CMTrace\Files”
Next we need to address changing the default program associated with a particular file extension on recent versions of Windows where the registry key is protected by a hash associated with the file type.
- Copy the PowerShell script below to “C:\Downloads\CMTrace\Files” & name it SFTA.ps1
- Script Reference: https://github.com/DanysysTeam/PS-SFTA
<# .SYNOPSIS Set File Type Association Windows 8/10/11 .DESCRIPTION Set File/Protocol Type Association Default Application Windows 8/10/11 .NOTES Version : 1.2.0 Author(s) : Danyfirex & Dany3j Credits : https://bbs.pediy.com/thread-213954.htm LMongrain - Hash Algorithm PureBasic Version License : MIT License Copyright : 2022 Danysys. <danysys.com> .EXAMPLE Get-FTA Show All Application Program Id .EXAMPLE Get-FTA .pdf Show Default Application Program Id for an Extension .EXAMPLE Set-FTA AcroExch.Document.DC .pdf Set Acrobat Reader DC as Default .pdf reader .EXAMPLE Set-FTA Applications\SumatraPDF.exe .pdf Set Sumatra PDF as Default .pdf reader .EXAMPLE Set-PTA ChromeHTML http Set Google Chrome as Default for http Protocol .EXAMPLE Register-FTA "C:\SumatraPDF.exe" .pdf -Icon "shell32.dll,100" Register Application and Set as Default for .pdf reader .LINK https://github.com/DanysysTeam/PS-SFTA #> function Get-FTA { [CmdletBinding()] param ( [Parameter(Mandatory = $false)] [String] $Extension ) if ($Extension) { Write-Verbose "Get File Type Association for $Extension" $assocFile = (Get-ItemProperty "HKCU:\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\$Extension\UserChoice" -ErrorAction SilentlyContinue).ProgId Write-Output $assocFile } else { Write-Verbose "Get File Type Association List" $assocList = Get-ChildItem HKCU:\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\* | ForEach-Object { $progId = (Get-ItemProperty "$($_.PSParentPath)\$($_.PSChildName)\UserChoice" -ErrorAction SilentlyContinue).ProgId if ($progId) { "$($_.PSChildName), $progId" } } Write-Output $assocList } } function Get-PTA { [CmdletBinding()] param ( [Parameter(Mandatory = $false)] [String] $Protocol ) if ($Protocol) { Write-Verbose "Get Protocol Type Association for $Protocol" $assocFile = (Get-ItemProperty "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\$Protocol\UserChoice" -ErrorAction SilentlyContinue).ProgId Write-Output $assocFile } else { Write-Verbose "Get Protocol Type Association List" $assocList = Get-ChildItem HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\* | ForEach-Object { $progId = (Get-ItemProperty "$($_.PSParentPath)\$($_.PSChildName)\UserChoice" -ErrorAction SilentlyContinue).ProgId if ($progId) { "$($_.PSChildName), $progId" } } Write-Output $assocList } } function Register-FTA { [CmdletBinding()] param ( [Parameter( Position = 0, Mandatory = $true)] [ValidateScript( { Test-Path $_ })] [String] $ProgramPath, [Parameter( Position = 1, Mandatory = $true)] [Alias("Protocol")] [String] $Extension, [Parameter( Position = 2, Mandatory = $false)] [String] $ProgId, [Parameter( Position = 3, Mandatory = $false)] [String] $Icon ) Write-Verbose "Register Application + Set Association" Write-Verbose "Application Path: $ProgramPath" if ($Extension.Contains(".")) { Write-Verbose "Extension: $Extension" } else { Write-Verbose "Protocol: $Extension" } if (!$ProgId) { $ProgId = "SFTA." + [System.IO.Path]::GetFileNameWithoutExtension($ProgramPath).replace(" ", "") + $Extension } $progCommand = """$ProgramPath"" ""%1""" Write-Verbose "ApplicationId: $ProgId" Write-Verbose "ApplicationCommand: $progCommand" try { $keyPath = "HKEY_CURRENT_USER\SOFTWARE\Classes\$Extension\OpenWithProgids" [Microsoft.Win32.Registry]::SetValue( $keyPath, $ProgId, ([byte[]]@()), [Microsoft.Win32.RegistryValueKind]::None) $keyPath = "HKEY_CURRENT_USER\SOFTWARE\Classes\$ProgId\shell\open\command" [Microsoft.Win32.Registry]::SetValue($keyPath, "", $progCommand) Write-Verbose "Register ProgId and ProgId Command OK" } catch { throw "Register ProgId and ProgId Command FAILED" } Set-FTA -ProgId $ProgId -Extension $Extension -Icon $Icon } function Remove-FTA { [CmdletBinding()] param ( [Parameter(Mandatory = $true)] [Alias("ProgId")] [String] $ProgramPath, [Parameter(Mandatory = $true)] [String] $Extension ) function local:Remove-UserChoiceKey { param ( [Parameter( Position = 0, Mandatory = $True )] [String] $Key ) $code = @' using System; using System.Runtime.InteropServices; using Microsoft.Win32; namespace Registry { public class Utils { [DllImport("advapi32.dll", SetLastError = true)] private static extern int RegOpenKeyEx(UIntPtr hKey, string subKey, int ulOptions, int samDesired, out UIntPtr hkResult); [DllImport("advapi32.dll", SetLastError=true, CharSet = CharSet.Unicode)] private static extern uint RegDeleteKey(UIntPtr hKey, string subKey); public static void DeleteKey(string key) { UIntPtr hKey = UIntPtr.Zero; RegOpenKeyEx((UIntPtr)0x80000001u, key, 0, 0x20019, out hKey); RegDeleteKey((UIntPtr)0x80000001u, key); } } } '@ try { Add-Type -TypeDefinition $code } catch {} try { [Registry.Utils]::DeleteKey($Key) } catch {} } function local:Update-Registry { $code = @' [System.Runtime.InteropServices.DllImport("Shell32.dll")] private static extern int SHChangeNotify(int eventId, int flags, IntPtr item1, IntPtr item2); public static void Refresh() { SHChangeNotify(0x8000000, 0, IntPtr.Zero, IntPtr.Zero); } '@ try { Add-Type -MemberDefinition $code -Namespace SHChange -Name Notify } catch {} try { [SHChange.Notify]::Refresh() } catch {} } if (Test-Path -Path $ProgramPath) { $ProgId = "SFTA." + [System.IO.Path]::GetFileNameWithoutExtension($ProgramPath).replace(" ", "") + $Extension } else { $ProgId = $ProgramPath } try { $keyPath = "Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\$Extension\UserChoice" Write-Verbose "Remove User UserChoice Key If Exist: $keyPath" Remove-UserChoiceKey $keyPath $keyPath = "HKCU:\SOFTWARE\Classes\$ProgId" Write-Verbose "Remove Key If Exist: $keyPath" Remove-Item -Path $keyPath -Recurse -ErrorAction Stop | Out-Null } catch { Write-Verbose "Key No Exist: $keyPath" } try { $keyPath = "HKCU:\SOFTWARE\Classes\$Extension\OpenWithProgids" Write-Verbose "Remove Property If Exist: $keyPath Property $ProgId" Remove-ItemProperty -Path $keyPath -Name $ProgId -ErrorAction Stop | Out-Null } catch { Write-Verbose "Property No Exist: $keyPath Property: $ProgId" } Update-Registry Write-Output "Removed: $ProgId" } function Set-FTA { [CmdletBinding()] param ( [Parameter(Mandatory = $true)] [String] $ProgId, [Parameter(Mandatory = $true)] [Alias("Protocol")] [String] $Extension, [String] $Icon, [switch] $DomainSID ) if (Test-Path -Path $ProgId) { $ProgId = "SFTA." + [System.IO.Path]::GetFileNameWithoutExtension($ProgId).replace(" ", "") + $Extension } Write-Verbose "ProgId: $ProgId" Write-Verbose "Extension/Protocol: $Extension" #Write required Application Ids to ApplicationAssociationToasts #When more than one application associated with an Extension/Protocol is installed ApplicationAssociationToasts need to be updated function local:Write-RequiredApplicationAssociationToasts { param ( [Parameter( Position = 0, Mandatory = $True )] [String] $ProgId, [Parameter( Position = 1, Mandatory = $True )] [String] $Extension ) try { $keyPath = "HKEY_CURRENT_USER\SOFTWARE\Microsoft\Windows\CurrentVersion\ApplicationAssociationToasts" [Microsoft.Win32.Registry]::SetValue($keyPath, $ProgId + "_" + $Extension, 0x0) Write-Verbose ("Write Reg ApplicationAssociationToasts OK: " + $ProgId + "_" + $Extension) } catch { Write-Verbose ("Write Reg ApplicationAssociationToasts FAILED: " + $ProgId + "_" + $Extension) } $allApplicationAssociationToasts = Get-ChildItem -Path HKLM:\SOFTWARE\Classes\$Extension\OpenWithList\* -ErrorAction SilentlyContinue | ForEach-Object { "Applications\$($_.PSChildName)" } $allApplicationAssociationToasts += @( ForEach ($item in (Get-ItemProperty -Path HKLM:\SOFTWARE\Classes\$Extension\OpenWithProgids -ErrorAction SilentlyContinue).PSObject.Properties ) { if ([string]::IsNullOrEmpty($item.Value) -and $item -ne "(default)") { $item.Name } }) $allApplicationAssociationToasts += Get-ChildItem -Path HKLM:SOFTWARE\Clients\StartMenuInternet\* , HKCU:SOFTWARE\Clients\StartMenuInternet\* -ErrorAction SilentlyContinue | ForEach-Object { (Get-ItemProperty ("$($_.PSPath)\Capabilities\" + (@("URLAssociations", "FileAssociations") | Select-Object -Index $Extension.Contains("."))) -ErrorAction SilentlyContinue).$Extension } $allApplicationAssociationToasts | ForEach-Object { if ($_) { if (Set-ItemProperty HKCU:\Software\Microsoft\Windows\CurrentVersion\ApplicationAssociationToasts $_"_"$Extension -Value 0 -Type DWord -ErrorAction SilentlyContinue -PassThru) { Write-Verbose ("Write Reg ApplicationAssociationToastsList OK: " + $_ + "_" + $Extension) } else { Write-Verbose ("Write Reg ApplicationAssociationToastsList FAILED: " + $_ + "_" + $Extension) } } } } function local:Update-RegistryChanges { $code = @' [System.Runtime.InteropServices.DllImport("Shell32.dll")] private static extern int SHChangeNotify(int eventId, int flags, IntPtr item1, IntPtr item2); public static void Refresh() { SHChangeNotify(0x8000000, 0, IntPtr.Zero, IntPtr.Zero); } '@ try { Add-Type -MemberDefinition $code -Namespace SHChange -Name Notify } catch {} try { [SHChange.Notify]::Refresh() } catch {} } function local:Set-Icon { param ( [Parameter( Position = 0, Mandatory = $True )] [String] $ProgId, [Parameter( Position = 1, Mandatory = $True )] [String] $Icon ) try { $keyPath = "HKEY_CURRENT_USER\SOFTWARE\Classes\$ProgId\DefaultIcon" [Microsoft.Win32.Registry]::SetValue($keyPath, "", $Icon) Write-Verbose "Write Reg Icon OK" Write-Verbose "Reg Icon: $keyPath" } catch { Write-Verbose "Write Reg Icon FAILED" } } function local:Write-ExtensionKeys { param ( [Parameter( Position = 0, Mandatory = $True )] [String] $ProgId, [Parameter( Position = 1, Mandatory = $True )] [String] $Extension, [Parameter( Position = 2, Mandatory = $True )] [String] $ProgHash ) function local:Remove-UserChoiceKey { param ( [Parameter( Position = 0, Mandatory = $True )] [String] $Key ) $code = @' using System; using System.Runtime.InteropServices; using Microsoft.Win32; namespace Registry { public class Utils { [DllImport("advapi32.dll", SetLastError = true)] private static extern int RegOpenKeyEx(UIntPtr hKey, string subKey, int ulOptions, int samDesired, out UIntPtr hkResult); [DllImport("advapi32.dll", SetLastError=true, CharSet = CharSet.Unicode)] private static extern uint RegDeleteKey(UIntPtr hKey, string subKey); public static void DeleteKey(string key) { UIntPtr hKey = UIntPtr.Zero; RegOpenKeyEx((UIntPtr)0x80000001u, key, 0, 0x20019, out hKey); RegDeleteKey((UIntPtr)0x80000001u, key); } } } '@ try { Add-Type -TypeDefinition $code } catch {} try { [Registry.Utils]::DeleteKey($Key) } catch {} } try { $keyPath = "Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\$Extension\UserChoice" Write-Verbose "Remove Extension UserChoice Key If Exist: $keyPath" Remove-UserChoiceKey $keyPath } catch { Write-Verbose "Extension UserChoice Key No Exist: $keyPath" } try { $keyPath = "HKEY_CURRENT_USER\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\$Extension\UserChoice" [Microsoft.Win32.Registry]::SetValue($keyPath, "Hash", $ProgHash) [Microsoft.Win32.Registry]::SetValue($keyPath, "ProgId", $ProgId) Write-Verbose "Write Reg Extension UserChoice OK" } catch { throw "Write Reg Extension UserChoice FAILED" } } function local:Write-ProtocolKeys { param ( [Parameter( Position = 0, Mandatory = $True )] [String] $ProgId, [Parameter( Position = 1, Mandatory = $True )] [String] $Protocol, [Parameter( Position = 2, Mandatory = $True )] [String] $ProgHash ) try { $keyPath = "HKCU:\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\$Protocol\UserChoice" Write-Verbose "Remove Protocol UserChoice Key If Exist: $keyPath" Remove-Item -Path $keyPath -Recurse -ErrorAction Stop | Out-Null } catch { Write-Verbose "Protocol UserChoice Key No Exist: $keyPath" } try { $keyPath = "HKEY_CURRENT_USER\Software\Microsoft\Windows\Shell\Associations\UrlAssociations\$Protocol\UserChoice" [Microsoft.Win32.Registry]::SetValue( $keyPath, "Hash", $ProgHash) [Microsoft.Win32.Registry]::SetValue($keyPath, "ProgId", $ProgId) Write-Verbose "Write Reg Protocol UserChoice OK" } catch { throw "Write Reg Protocol UserChoice FAILED" } } function local:Get-UserExperience { [OutputType([string])] $userExperienceSearch = "User Choice set via Windows User Experience" $user32Path = [Environment]::GetFolderPath([Environment+SpecialFolder]::SystemX86) + "\Shell32.dll" $fileStream = [System.IO.File]::Open($user32Path, [System.IO.FileMode]::Open, [System.IO.FileAccess]::Read, [System.IO.FileShare]::ReadWrite) $binaryReader = New-Object System.IO.BinaryReader($fileStream) [Byte[]] $bytesData = $binaryReader.ReadBytes(5mb) $fileStream.Close() $dataString = [Text.Encoding]::Unicode.GetString($bytesData) $position1 = $dataString.IndexOf($userExperienceSearch) $position2 = $dataString.IndexOf("}", $position1) Write-Output $dataString.Substring($position1, $position2 - $position1 + 1) } function local:Get-UserSid { [OutputType([string])] $userSid = ((New-Object System.Security.Principal.NTAccount([Environment]::UserName)).Translate([System.Security.Principal.SecurityIdentifier]).value).ToLower() Write-Output $userSid } #use in this special case #https://github.com/DanysysTeam/PS-SFTA/pull/7 function local:Get-UserSidDomain { if (-not ("System.DirectoryServices.AccountManagement" -as [type])) { Add-Type -AssemblyName System.DirectoryServices.AccountManagement } [OutputType([string])] $userSid = ([System.DirectoryServices.AccountManagement.UserPrincipal]::Current).SID.Value.ToLower() Write-Output $userSid } function local:Get-HexDateTime { [OutputType([string])] $now = [DateTime]::Now $dateTime = [DateTime]::New($now.Year, $now.Month, $now.Day, $now.Hour, $now.Minute, 0) $fileTime = $dateTime.ToFileTime() $hi = ($fileTime -shr 32) $low = ($fileTime -band 0xFFFFFFFFL) $dateTimeHex = ($hi.ToString("X8") + $low.ToString("X8")).ToLower() Write-Output $dateTimeHex } function Get-Hash { [CmdletBinding()] param ( [Parameter( Position = 0, Mandatory = $True )] [string] $BaseInfo ) function local:Get-ShiftRight { [CmdletBinding()] param ( [Parameter( Position = 0, Mandatory = $true)] [long] $iValue, [Parameter( Position = 1, Mandatory = $true)] [int] $iCount ) if ($iValue -band 0x80000000) { Write-Output (( $iValue -shr $iCount) -bxor 0xFFFF0000) } else { Write-Output ($iValue -shr $iCount) } } function local:Get-Long { [CmdletBinding()] param ( [Parameter( Position = 0, Mandatory = $true)] [byte[]] $Bytes, [Parameter( Position = 1)] [int] $Index = 0 ) Write-Output ([BitConverter]::ToInt32($Bytes, $Index)) } function local:Convert-Int32 { param ( [Parameter( Position = 0, Mandatory = $true)] $Value ) [byte[]] $bytes = [BitConverter]::GetBytes($Value) return [BitConverter]::ToInt32( $bytes, 0) } [Byte[]] $bytesBaseInfo = [System.Text.Encoding]::Unicode.GetBytes($baseInfo) $bytesBaseInfo += 0x00, 0x00 $MD5 = New-Object -TypeName System.Security.Cryptography.MD5CryptoServiceProvider [Byte[]] $bytesMD5 = $MD5.ComputeHash($bytesBaseInfo) $lengthBase = ($baseInfo.Length * 2) + 2 $length = (($lengthBase -band 4) -le 1) + (Get-ShiftRight $lengthBase 2) - 1 $base64Hash = "" if ($length -gt 1) { $map = @{PDATA = 0; CACHE = 0; COUNTER = 0 ; INDEX = 0; MD51 = 0; MD52 = 0; OUTHASH1 = 0; OUTHASH2 = 0; R0 = 0; R1 = @(0, 0); R2 = @(0, 0); R3 = 0; R4 = @(0, 0); R5 = @(0, 0); R6 = @(0, 0); R7 = @(0, 0) } $map.CACHE = 0 $map.OUTHASH1 = 0 $map.PDATA = 0 $map.MD51 = (((Get-Long $bytesMD5) -bor 1) + 0x69FB0000L) $map.MD52 = ((Get-Long $bytesMD5 4) -bor 1) + 0x13DB0000L $map.INDEX = Get-ShiftRight ($length - 2) 1 $map.COUNTER = $map.INDEX + 1 while ($map.COUNTER) { $map.R0 = Convert-Int32 ((Get-Long $bytesBaseInfo $map.PDATA) + [long]$map.OUTHASH1) $map.R1[0] = Convert-Int32 (Get-Long $bytesBaseInfo ($map.PDATA + 4)) $map.PDATA = $map.PDATA + 8 $map.R2[0] = Convert-Int32 (($map.R0 * ([long]$map.MD51)) - (0x10FA9605L * ((Get-ShiftRight $map.R0 16)))) $map.R2[1] = Convert-Int32 ((0x79F8A395L * ([long]$map.R2[0])) + (0x689B6B9FL * (Get-ShiftRight $map.R2[0] 16))) $map.R3 = Convert-Int32 ((0xEA970001L * $map.R2[1]) - (0x3C101569L * (Get-ShiftRight $map.R2[1] 16) )) $map.R4[0] = Convert-Int32 ($map.R3 + $map.R1[0]) $map.R5[0] = Convert-Int32 ($map.CACHE + $map.R3) $map.R6[0] = Convert-Int32 (($map.R4[0] * [long]$map.MD52) - (0x3CE8EC25L * (Get-ShiftRight $map.R4[0] 16))) $map.R6[1] = Convert-Int32 ((0x59C3AF2DL * $map.R6[0]) - (0x2232E0F1L * (Get-ShiftRight $map.R6[0] 16))) $map.OUTHASH1 = Convert-Int32 ((0x1EC90001L * $map.R6[1]) + (0x35BD1EC9L * (Get-ShiftRight $map.R6[1] 16))) $map.OUTHASH2 = Convert-Int32 ([long]$map.R5[0] + [long]$map.OUTHASH1) $map.CACHE = ([long]$map.OUTHASH2) $map.COUNTER = $map.COUNTER - 1 } [Byte[]] $outHash = @(0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00) [byte[]] $buffer = [BitConverter]::GetBytes($map.OUTHASH1) $buffer.CopyTo($outHash, 0) $buffer = [BitConverter]::GetBytes($map.OUTHASH2) $buffer.CopyTo($outHash, 4) $map = @{PDATA = 0; CACHE = 0; COUNTER = 0 ; INDEX = 0; MD51 = 0; MD52 = 0; OUTHASH1 = 0; OUTHASH2 = 0; R0 = 0; R1 = @(0, 0); R2 = @(0, 0); R3 = 0; R4 = @(0, 0); R5 = @(0, 0); R6 = @(0, 0); R7 = @(0, 0) } $map.CACHE = 0 $map.OUTHASH1 = 0 $map.PDATA = 0 $map.MD51 = ((Get-Long $bytesMD5) -bor 1) $map.MD52 = ((Get-Long $bytesMD5 4) -bor 1) $map.INDEX = Get-ShiftRight ($length - 2) 1 $map.COUNTER = $map.INDEX + 1 while ($map.COUNTER) { $map.R0 = Convert-Int32 ((Get-Long $bytesBaseInfo $map.PDATA) + ([long]$map.OUTHASH1)) $map.PDATA = $map.PDATA + 8 $map.R1[0] = Convert-Int32 ($map.R0 * [long]$map.MD51) $map.R1[1] = Convert-Int32 ((0xB1110000L * $map.R1[0]) - (0x30674EEFL * (Get-ShiftRight $map.R1[0] 16))) $map.R2[0] = Convert-Int32 ((0x5B9F0000L * $map.R1[1]) - (0x78F7A461L * (Get-ShiftRight $map.R1[1] 16))) $map.R2[1] = Convert-Int32 ((0x12CEB96DL * (Get-ShiftRight $map.R2[0] 16)) - (0x46930000L * $map.R2[0])) $map.R3 = Convert-Int32 ((0x1D830000L * $map.R2[1]) + (0x257E1D83L * (Get-ShiftRight $map.R2[1] 16))) $map.R4[0] = Convert-Int32 ([long]$map.MD52 * ([long]$map.R3 + (Get-Long $bytesBaseInfo ($map.PDATA - 4)))) $map.R4[1] = Convert-Int32 ((0x16F50000L * $map.R4[0]) - (0x5D8BE90BL * (Get-ShiftRight $map.R4[0] 16))) $map.R5[0] = Convert-Int32 ((0x96FF0000L * $map.R4[1]) - (0x2C7C6901L * (Get-ShiftRight $map.R4[1] 16))) $map.R5[1] = Convert-Int32 ((0x2B890000L * $map.R5[0]) + (0x7C932B89L * (Get-ShiftRight $map.R5[0] 16))) $map.OUTHASH1 = Convert-Int32 ((0x9F690000L * $map.R5[1]) - (0x405B6097L * (Get-ShiftRight ($map.R5[1]) 16))) $map.OUTHASH2 = Convert-Int32 ([long]$map.OUTHASH1 + $map.CACHE + $map.R3) $map.CACHE = ([long]$map.OUTHASH2) $map.COUNTER = $map.COUNTER - 1 } $buffer = [BitConverter]::GetBytes($map.OUTHASH1) $buffer.CopyTo($outHash, 8) $buffer = [BitConverter]::GetBytes($map.OUTHASH2) $buffer.CopyTo($outHash, 12) [Byte[]] $outHashBase = @(0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00) $hashValue1 = ((Get-Long $outHash 8) -bxor (Get-Long $outHash)) $hashValue2 = ((Get-Long $outHash 12) -bxor (Get-Long $outHash 4)) $buffer = [BitConverter]::GetBytes($hashValue1) $buffer.CopyTo($outHashBase, 0) $buffer = [BitConverter]::GetBytes($hashValue2) $buffer.CopyTo($outHashBase, 4) $base64Hash = [Convert]::ToBase64String($outHashBase) } Write-Output $base64Hash } Write-Verbose "Getting Hash For $ProgId $Extension" If ($DomainSID.IsPresent) { Write-Verbose "Use Get-UserSidDomain" } Else { Write-Verbose "Use Get-UserSid" } $userSid = If ($DomainSID.IsPresent) { Get-UserSidDomain } Else { Get-UserSid } $userExperience = Get-UserExperience $userDateTime = Get-HexDateTime Write-Debug "UserDateTime: $userDateTime" Write-Debug "UserSid: $userSid" Write-Debug "UserExperience: $userExperience" $baseInfo = "$Extension$userSid$ProgId$userDateTime$userExperience".ToLower() Write-Verbose "baseInfo: $baseInfo" $progHash = Get-Hash $baseInfo Write-Verbose "Hash: $progHash" #Write AssociationToasts List Write-RequiredApplicationAssociationToasts $ProgId $Extension #Handle Extension Or Protocol if ($Extension.Contains(".")) { Write-Verbose "Write Registry Extension: $Extension" Write-ExtensionKeys $ProgId $Extension $progHash } else { Write-Verbose "Write Registry Protocol: $Extension" Write-ProtocolKeys $ProgId $Extension $progHash } if ($Icon) { Write-Verbose "Set Icon: $Icon" Set-Icon $ProgId $Icon } Update-RegistryChanges } function Set-PTA { [CmdletBinding()] param ( [Parameter(Mandatory = $true)] [String] $ProgId, [Parameter(Mandatory = $true)] [String] $Protocol, [String] $Icon ) Set-FTA -ProgId $ProgId -Protocol $Protocol -Icon $Icon }
Next we need to create a batch script that will run once for each new user profile in order to set CMTrace as the default app for log files.
- Open Notepad or your favorite text editor
- Add the following lines:
cd "C:\ProgramData\SFTA" PowerShell.exe -ExecutionPolicy Bypass -Command "& { . .\SFTA.ps1; Register-FTA 'C:\Windows\CMTrace.exe' '.log' -Verbose }" (goto) 2>nul & del "%~f0"
- Save the file to “C:\Downloads\CMTrace\Files” and name it: Register-CMTrace.cmd
- You should now see the following files inside the Files directory
- CMTrace.exe
- SFTA.ps1
- Register-CMTrace.cmd
- You should now see the following files inside the Files directory
- Copy the PowerShell script below to “C:\Downloads\CMTrace“ & name it Deploy-CMTrace.ps1
<# .SYNOPSIS This script performs the installation or uninstallation of CMTrace. # LICENSE # PowerShell App Deployment Toolkit - Provides a set of functions to perform common application deployment tasks on Windows. Copyright (C) 2017 - Sean Lillis, Dan Cunningham, Muhammad Mashwani, Aman Motazedian. This program is free software: you can redistribute it and/or modify it under the terms of the GNU Lesser General Public License as published by the Free Software Foundation, either version 3 of the License, or any later version. This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a copy of the GNU Lesser General Public License along with this program. If not, see <http://www.gnu.org/licenses/>. .DESCRIPTION The script is provided as a template to perform an install or uninstall of an application(s). The script either performs an "Install" deployment type or an "Uninstall" deployment type. The install deployment type is broken down into 3 main sections/phases: Pre-Install, Install, and Post-Install. The script dot-sources the AppDeployToolkitMain.ps1 script which contains the logic and functions required to install or uninstall an application. .PARAMETER DeploymentType The type of deployment to perform. Default is: Install. .PARAMETER DeployMode Specifies whether the installation should be run in Interactive, Silent, or NonInteractive mode. Default is: Interactive. Options: Interactive = Shows dialogs, Silent = No dialogs, NonInteractive = Very silent, i.e. no blocking apps. NonInteractive mode is automatically set if it is detected that the process is not user interactive. .PARAMETER AllowRebootPassThru Allows the 3010 return code (requires restart) to be passed back to the parent process (e.g. SCCM) if detected from an installation. If 3010 is passed back to SCCM, a reboot prompt will be triggered. .PARAMETER TerminalServerMode Changes to "user install mode" and back to "user execute mode" for installing/uninstalling applications for Remote Destkop Session Hosts/Citrix servers. .PARAMETER DisableLogging Disables logging to file for the script. Default is: $false. .EXAMPLE PowerShell.exe .\Deploy-CMTrace.ps1 -DeploymentType "Install" -DeployMode "NonInteractive" .EXAMPLE PowerShell.exe .\Deploy-CMTrace.ps1 -DeploymentType "Install" -DeployMode "Silent" .EXAMPLE PowerShell.exe .\Deploy-CMTrace.ps1 -DeploymentType "Install" -DeployMode "Interactive" .EXAMPLE PowerShell.exe .\Deploy-CMTrace.ps1 -DeploymentType "Uninstall" -DeployMode "NonInteractive" .EXAMPLE PowerShell.exe .\Deploy-CMTrace.ps1 -DeploymentType "Uninstall" -DeployMode "Silent" .EXAMPLE PowerShell.exe .\Deploy-CMTrace.ps1 -DeploymentType "Uninstall" -DeployMode "Interactive" .NOTES Toolkit Exit Code Ranges: 60000 - 68999: Reserved for built-in exit codes in Deploy-Application.ps1, Deploy-Application.exe, and AppDeployToolkitMain.ps1 69000 - 69999: Recommended for user customized exit codes in Deploy-Application.ps1 70000 - 79999: Recommended for user customized exit codes in AppDeployToolkitExtensions.ps1 .LINK http://psappdeploytoolkit.com #> [CmdletBinding()] Param ( [Parameter(Mandatory=$false)] [ValidateSet('Install','Uninstall','Repair')] [string]$DeploymentType = 'Install', [Parameter(Mandatory=$false)] [ValidateSet('Interactive','Silent','NonInteractive')] [string]$DeployMode = 'Interactive', [Parameter(Mandatory=$false)] [switch]$AllowRebootPassThru = $false, [Parameter(Mandatory=$false)] [switch]$TerminalServerMode = $false, [Parameter(Mandatory=$false)] [switch]$DisableLogging = $false ) Try { ## Set the script execution policy for this process Try { Set-ExecutionPolicy -ExecutionPolicy 'ByPass' -Scope 'Process' -Force -ErrorAction 'Stop' } Catch {} ##*=============================================== ##* VARIABLE DECLARATION ##*=============================================== ## Variables: Application [string]$appVendor = '' [string]$appName = 'CMTrace' [string]$appVersion = '' [string]$appArch = '' [string]$appLang = '' [string]$appRevision = '' [string]$appScriptVersion = '1.0.0' [string]$appScriptDate = 'XX/XX/20XX' [string]$appScriptAuthor = 'Jason Bergner' ##*=============================================== ## Variables: Install Titles (Only set here to override defaults set by the toolkit) [string]$installName = '' [string]$installTitle = 'CMTrace' ##* Do not modify section below #region DoNotModify ## Variables: Exit Code [int32]$mainExitCode = 0 ## Variables: Script [string]$deployAppScriptFriendlyName = 'Deploy Application' [version]$deployAppScriptVersion = [version]'3.8.4' [string]$deployAppScriptDate = '26/01/2021' [hashtable]$deployAppScriptParameters = $psBoundParameters ## Variables: Environment If (Test-Path -LiteralPath 'variable:HostInvocation') { $InvocationInfo = $HostInvocation } Else { $InvocationInfo = $MyInvocation } [string]$scriptDirectory = Split-Path -Path $InvocationInfo.MyCommand.Definition -Parent ## Dot source the required App Deploy Toolkit Functions Try { [string]$moduleAppDeployToolkitMain = "$scriptDirectory\AppDeployToolkit\AppDeployToolkitMain.ps1" If (-not (Test-Path -LiteralPath $moduleAppDeployToolkitMain -PathType 'Leaf')) { Throw "Module does not exist at the specified location [$moduleAppDeployToolkitMain]." } If ($DisableLogging) { . $moduleAppDeployToolkitMain -DisableLogging } Else { . $moduleAppDeployToolkitMain } } Catch { If ($mainExitCode -eq 0){ [int32]$mainExitCode = 60008 } Write-Error -Message "Module [$moduleAppDeployToolkitMain] failed to load: `n$($_.Exception.Message)`n `n$($_.InvocationInfo.PositionMessage)" -ErrorAction 'Continue' ## Exit the script, returning the exit code to SCCM If (Test-Path -LiteralPath 'variable:HostInvocation') { $script:ExitCode = $mainExitCode; Exit } Else { Exit $mainExitCode } } #endregion ##* Do not modify section above ##*=============================================== ##* END VARIABLE DECLARATION ##*=============================================== If ($deploymentType -ine 'Uninstall' -and $deploymentType -ine 'Repair') { ##*=============================================== ##* PRE-INSTALLATION ##*=============================================== [string]$installPhase = 'Pre-Installation' ## Show Welcome Message, Close CMTrace With a 60 Second Countdown Before Automatically Closing Show-InstallationWelcome -CloseApps 'CMTrace' -CloseAppsCountdown 60 ## Show Progress Message (with the default message) Show-InstallationProgress ## Remove Any Existing CMTrace from C:\Windows Directory (If Present) $CMTrace = "$envWinDir\CMTrace.exe" If (Test-Path $CMTrace) { Write-Log -Message "Removing CMTrace from C:\Windows Directory." Remove-Item -Path "$CMTrace" -Force -ErrorAction SilentlyContinue Sleep -Seconds 5 } ##*=============================================== ##* INSTALLATION ##*=============================================== [string]$installPhase = 'Installation' ## Install CMTrace $ExePath = Get-ChildItem -Path "$dirFiles" -Include CMTrace.exe -File -Recurse -ErrorAction SilentlyContinue If($ExePath.Exists) { Write-Log -Message "Found $($ExePath.FullName), now attempting to copy $installTitle to the Windows directory." Show-InstallationProgress "Installing CMTrace. This may take some time. Please wait..." Copy-Item $ExePath -Destination "$envWinDir" -ErrorAction SilentlyContinue Sleep -Seconds 5 ## Create CMTrace Start Menu Shortcut (Optional) Write-Log -Message "Creating CMTrace Start Menu Shortcut." -Source $deployAppScriptFriendlyName $TargetFile = "$envWinDir\CMTrace.exe" $ShortcutFile = "$envAllUsersProfile\Microsoft\Windows\Start Menu\Programs\CMTrace.lnk" $WScriptShell = New-Object -ComObject WScript.Shell $Shortcut = $WScriptShell.CreateShortcut($ShortcutFile) $Shortcut.TargetPath = $TargetFile $Shortcut.Save() ## Set CMTrace As Default Log Viewer Write-Log -Message "Setting CMTrace As Default Log Viewer." Set-RegistryKey -Key 'HKLM\Software\Classes\.lo_' -Name '(Default)' -Value "Log.File" -Type String Set-RegistryKey -Key 'HKLM\Software\Classes\.log' -Name '(Default)' -Value "Log.File" -Type String Set-RegistryKey -Key 'HKLM\Software\Classes\Log.File\shell\open\command' -Name '(Default)' -Value "`"C:\Windows\CMTrace.exe`" `"%1`"" -Type String Set-RegistryKey -Key 'HKLM\Software\Microsoft\Trace32' [scriptblock]$HKCURegistrySettings = { Set-RegistryKey -Key 'HKCU\Software\Microsoft\Trace32' -Name 'Register File Types' -Value 0 -Type String -SID $UserProfile.SID } Invoke-HKCURegistrySettingsForAllUsers -RegistrySettings $HKCURegistrySettings -ErrorAction SilentlyContinue ## Set CMTrace As Default App For Log Files Write-Log -Message "Setting CMTrace As Default App For Log Files." [scriptblock]$HKCURegistrySettings = { Remove-RegistryKey -Key 'HKCU\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\.log' -Recurse -SID $UserProfile.SID Set-RegistryKey -Key 'HKCU\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\.log\OpenWithList' -Name 'a' -Value 'CMTrace.exe' -Type String -SID $UserProfile.SID Set-RegistryKey -Key 'HKCU\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\.log\OpenWithList' -Name 'MRUList' -Value 'a' -Type String -SID $UserProfile.SID Set-RegistryKey -Key 'HKCU\Software\Microsoft\Windows\CurrentVersion\Explorer\FileExts\.log\OpenWithProgids' -Name 'Log.File' -Type Binary -SID $UserProfile.SID } Invoke-HKCURegistrySettingsForAllUsers -RegistrySettings $HKCURegistrySettings -ErrorAction SilentlyContinue $output = Execute-Process -Path "$envSystem32Directory\cmd.exe" -Parameters '/c Ftype Log.File=C:\Windows\CMTrace.exe %1' -WindowStyle Hidden -PassThru Write-Log $output.StdOut ## Set CMTrace As Default App For Log Files for New User Profiles $SFTA = Get-ChildItem -Path "$dirFiles" -Include SFTA.ps1 -File -Recurse -ErrorAction SilentlyContinue If($SFTA.Exists) { $FTA = Get-ChildItem -Path "$dirFiles" -Include Register-CMTrace.cmd -File -Recurse -ErrorAction SilentlyContinue If($FTA.Exists) { Write-Log -Message "Setting CMTrace As Default App For Log Files for New User Profiles." New-Item "$envAllUsersProfile\SFTA\" -ItemType Directory -Force Copy-Item $SFTA -Destination "$envAllUsersProfile\SFTA\" -ErrorAction SilentlyContinue $Default = "C:\Users\Default\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Startup\" New-Item "$Default" -ItemType Directory -Force Copy-Item $FTA -Destination "$Default" -ErrorAction SilentlyContinue } } } ##*=============================================== ##* POST-INSTALLATION ##*=============================================== [string]$installPhase = 'Post-Installation' } ElseIf ($deploymentType -ieq 'Uninstall') { ##*=============================================== ##* PRE-UNINSTALLATION ##*=============================================== [string]$installPhase = 'Pre-Uninstallation' ## Show Welcome Message, Close CMTrace With a 60 Second Countdown Before Automatically Closing Show-InstallationWelcome -CloseApps 'CMTrace' -CloseAppsCountdown 60 ## Show Progress Message (With a Message to Indicate the Application is Being Uninstalled) Show-InstallationProgress -StatusMessage "Uninstalling the $installTitle Application. Please Wait..." ##*=============================================== ##* UNINSTALLATION ##*=============================================== [string]$installPhase = 'Uninstallation' ## Remove Any Existing CMTrace from C:\Windows Directory (If Present) $CMTrace = "$envWinDir\CMTrace.exe" If (Test-Path $CMTrace) { Write-Log -Message "Removing CMTrace from C:\Windows Directory." Remove-Item -Path "$CMTrace" -Force -ErrorAction SilentlyContinue Sleep -Seconds 5 ## Reset Notepad as Default Log Viewer Write-Log -Message "Resetting Notepad as Default Log Viewer." Set-RegistryKey -Key 'HKLM\Software\Classes\Log.File\shell\open\command' -Name '(Default)' -Value "`"C:\Windows\Notepad.exe`" `"%1`"" -Type String ## Remove Trace32 Registry Key from HKCU for All Users [scriptblock]$HKCURegistrySettings = { Write-Log -Message "Removing Trace32 Registry Key from HKCU for All Users." Remove-RegistryKey -Key 'HKCU\Software\Microsoft\Trace32' -Recurse -SID $UserProfile.SID } Invoke-HKCURegistrySettingsForAllUsers -RegistrySettings $HKCURegistrySettings -ErrorAction SilentlyContinue ## Reset Notepad As Default App For Log Files Write-Log -Message "Resetting Notepad As Default App For Log Files." $output = Execute-Process -Path "$envSystem32Directory\cmd.exe" -Parameters '/c Ftype Log.File=C:\Windows\Notepad.exe %1' -WindowStyle Hidden -PassThru Write-Log $output.StdOut } ## Remove Register-CMTrace.cmd Script From Default Profile StartUp Folder If (Test-Path -Path "C:\Users\Default\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Startup\Register-CMTrace.cmd" ) { Write-Log -Message "Removing Register-CMTrace.cmd Script From Default Profile StartUp Folder." Remove-Item -Path "C:\Users\Default\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Startup\Register-CMTrace.cmd" -Force -Recurse -ErrorAction SilentlyContinue Sleep -Seconds 5 } ## Remove CMTrace Start Menu Shortcut (If Present) If (Test-Path -Path "$envAllUsersProfile\Microsoft\Windows\Start Menu\Programs\CMTrace.lnk") { Write-Log -Message "Removing the CMTrace Start Menu Shortcut." Remove-Item -Path "$envAllUsersProfile\Microsoft\Windows\Start Menu\Programs\CMTrace.lnk" -Force -Recurse -ErrorAction SilentlyContinue Sleep -Seconds 5 } ##*=============================================== ##* POST-UNINSTALLATION ##*=============================================== [string]$installPhase = 'Post-Uninstallation' } ElseIf ($deploymentType -ieq 'Repair') { ##*=============================================== ##* PRE-REPAIR ##*=============================================== [string]$installPhase = 'Pre-Repair' ##*=============================================== ##* REPAIR ##*=============================================== [string]$installPhase = 'Repair' ##*=============================================== ##* POST-REPAIR ##*=============================================== [string]$installPhase = 'Post-Repair' } ##*=============================================== ##* END SCRIPT BODY ##*=============================================== ## Call the Exit-Script function to perform final cleanup operations Exit-Script -ExitCode $mainExitCode } Catch { [int32]$mainExitCode = 60001 [string]$mainErrorMessage = "$(Resolve-Error)" Write-Log -Message $mainErrorMessage -Severity 3 -Source $deployAppScriptFriendlyName Show-DialogBox -Text $mainErrorMessage -Icon 'Stop' Exit-Script -ExitCode $mainExitCode }
Ok, all the hard work is done and now you can install & set CMTrace as default log viewer for all users using one single PowerShell script. Simply change the DeploymentType parameter to install or uninstall. Logging functionality is built-in automatically and you can view the log files under “C:\Windows\Logs\Software”.
Install & Set CMTrace as Default Log Viewer for All Users NonInteractive Install (PowerShell)
NonInteractive means Very Silent, i.e. no blocking apps. This is automatically set if it is detected that the process is not running in the user session and it is not possible for anyone to provide input using a mouse or keyboard.
- Open Windows PowerShell by Right-Clicking on Windows PowerShell and selecting Run as Administrator
- Change the directory to “C:\Downloads\CMTrace”
- PS C:\Downloads\CMTrace>
- Enter the following command:
Powershell.exe -ExecutionPolicy Bypass .\Deploy-CMTrace.ps1 -DeploymentType "Install" -DeployMode "NonInteractive"
Install & Set CMTrace as Default Log Viewer for All Users Silent Install (PowerShell)
Silent means no dialogs (progress and balloon tip notifications are suppressed).
- Open Windows PowerShell by Right-Clicking on Windows PowerShell and selecting Run as Administrator
- Change the directory to “C:\Downloads\CMTrace“
- PS C:\Downloads\CMTrace>
- Enter the following command:
Powershell.exe -ExecutionPolicy Bypass .\Deploy-CMTrace.ps1 -DeploymentType "Install" -DeployMode "Silent"
Install & Set CMTrace as Default Log Viewer for All Users Interactive Install (PowerShell)
Interactive means the install will show dialogs including progress and balloon tip notifications.
- Open Windows PowerShell by Right-Clicking on Windows PowerShell and selecting Run as Administrator
- Change the directory to “C:\Downloads\CMTrace“
- PS C:\Downloads\CMTrace>
- Enter the following command:
Powershell.exe -ExecutionPolicy Bypass .\Deploy-CMTrace.ps1 -DeploymentType "Install" -DeployMode "Interactive"
Always make sure to test everything in a development environment prior to implementing anything into production. The information in this article is provided “As Is” without warranty of any kind.